One of my favorite features of AngularJS is the use of HTML attributes to apply controllers and directives directly to your DOM elements. Why is this so useful?
- It is intuitive for developers to discover what code is being applied to elements.
- It enables generic registration, removing boiler plate document ready methods.
- It provides hierarchical scope, encouraging single responsibility controls.
jQuery plugins are already designed to be applied to collections of elements, so let's just add the ability to dynamically apply plugins via HTML attributes! This is how we can make jQuery a bit more Angular.
Sample Script
Our sample jQuery plugin is super simple; it just makes an element fade in and out continuously. This is a very simple behavior, but the point is that it is just a jQuery plugin!
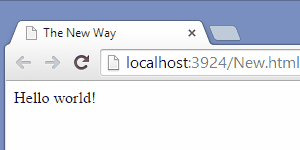
(function ($) {
$.fn.blink = function() {
var $el = this;
setInterval(blinkEl, 1000);
function blinkEl() {
$el.fadeToggle();
}
};
})(jQuery);